Understanding Binary Tree And Lowest Common Ancestor
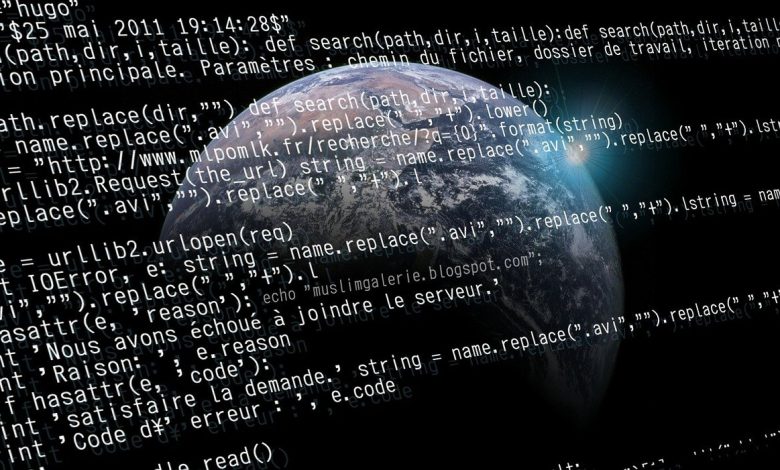
“Finding the lowest common ancestry with a given binary tree” obviously needs thorough understanding of the concepts.
That’s why we have compiled this piece of information for you!
A binary tree essentially consists of nodes that store a certain amount of data. Each of these node may or may not have extended children nodes. In the case of a binary tree (BT), each node can only possess two extended children nodes.
If you would like to learn more about the BT, the Ica of binary tree, and how to find the kth smallest element in a sorted matrix, then keep on reading.
Binary Tree
What is a binary tree?
It is a graph data structure in which each node has no more than two offspring. These are referred to as the left child and the right child. The top node of a tree is called the Root node.
Imagine a data structure in the form of a conceptual tree which has extended nodes. Also imagine, you can trace each node back to the first node (the root of the tree). That is how you would describe the structure of a BT.
While studying the BT you have to jot down a few common phrases or words. These we use in the context of a binary tree such as Ancestor, Parent node, Children, Leaf, etc.
In the old programming books, the binary tree has also been referred to as the rooted binary tree. This was to shed light on the fact that the tree is always rooted.
Now, in the field of programming, it is used for sorting, searching data, and for other applications. Navigating a BT is much easier than any other form of data structure. This is because you can trace any given node back to its parent node. You can also trace it further back to the first ancestor node, referred to as the root of the binary tree.
The following are some of the most common terms used in this context:
- Children: Every parent node of the tree has two children or less but never more than two.
- Left child: The left node of a parent node is known as the left child of the node.
- Right child: Similarly the right node of a parent node is known as the right child of the node.
- Sub tree: If you look at the structure of the tree it will always have a parent node and one or two children nodes. These nodes further branch out into other children nodes. Hence forming a sub-tree branch of the main binary tree.
- Ancestor: The ancestor of any given node of a tree is the predecessor of its parent node till the last applicable node.
These were some of the basics of a BT that you have to keep in mind in order to navigate the whole data structure.
LCA of binary tree
LCA of binary tree which is also known as the lowest common ancestor of a binary tree can be explained as the common ancestor of two given nodes (for example n4 and n5) which is the farthest from the root of the tree.
This means that if you look at the diagram of a common binary tree where n1 is the root, n2 and n3 are the left and right children nodes of n1, and finally, n2 further has n4 and n5 as the left and right children nodes.
You will be able to comprehend that n2 is the lowest common ancestor node for nodes n4 and n5 respectively.
This also means that n2 is the parent node of n4 and n5 which also makes it the lowest common ancestor of the two nodes.
As we have discussed above in the definition of ancestor nodes we have already explained that these nodes can be traced through the parent node of any given node till we reach the root of the tree.
The same formula applies when we are trying to figure out the lowest common ancestor of a binary tree which in short is also referred to as the LCA of binary tree.
This is not to be confused with the ultimate root or the parent node of the tree, if you encounter any problem related to this concept you will only have to figure out the parent node of the given pair of children nodes.
What is the Bottom view of a binary tree?
Only the nodes of the binary tree that are visible when viewed from the bottom are printed in its bottom view.
The binary tree’s bottom view is the horizontal distance between the bottommost nodes.
The horizontal distance between nodes in a binary tree is defined as follows:
- The horizontal distance of the root from itself is 0. For the right child of the root node it is +1. For the left child of the root node it is -1.
- If node ‘n’ is the right child of its parent. Then its horizontal distance from root equals its parent’s horizontal distance from root + 1.
- If node ‘n’ is the left child of its parent. Then its horizontal distance from the root equals its parent’s horizontal distance from root – 1.
- If more than one node is at the same horizontal distance and is the bottom-most node for that horizontal distance, including the one on the right.
Now, moving on we will discuss the kth smallest element in a sorted matrix.
Finding the kth smallest element in a given sorted matrix
In order to do this, you can execute the functions of the “queue”. This can be done by finding out the minimum & maximum element of the matrix. This is followed by implying the priority queue.
This algorithm involves sorting of n square elements. So the time complexity would be in the order of n square logs of n square. It is a bit time-consuming but it will definitely get the job done.
Let’s move on to the last topic of our discussion in this blog which is related to the lowest common ancestor in a binary tree.
Lowest Common Ancestor
LCA in a binary tree is one of the many applications of the concept used in computer science. In the simplest terms, it is mainly the root of the tree.
This is the first parent node which consists of two children nodes and further extends to create many sub-trees. This node can be traced back from any given node on the tree. Therefore the root node is referred to as the LCA of a binary tree.
Conclusion
Let us conclude what we have discussed so far and what are the key takeaways from this blog. Refer to the structure of the tree, to understand the example provided in this blog.
Next up, we have also discussed what is the lowest common ancestor of a BT. And also how you can trace the node, which in other terms is also known as the LCA of binary tree.
And lastly, we have discussed a sample problem to make you understand how to figure out the kth smallest element in a sorted matrix.
We hope this blog was able to clear most of your doubts about the Binary Tree & the LCA.
Click here to read – The advantages of learning Java programming language in 2021
Happy Learning